Overview
Authentication SDK is a Unity package to handle login to STREAMSIX platform.
The platform allows user to create an account on the STREAMSIX platform, or link to an account from a 3rd party OIDC provider (such as Twitch, Apple, etc)
Initialization
Call AuthController.InitAsync()
before using any other functions in the Auth package.
Login Flow
There are serveral ways to login:
Login through STREAMSIX website
Login through STREAMSIX by username and password. (Not recommended due to security concern)
Login to 3rd party OIDC (such as Twitch), use the obtained access token to login to STREAMSIX.
Login to STREAMSIX, login to 3rd party OIDC, then link the two account.
Login through STREAMSIX website
Call AuthController.LoginExternalWebpage()
to launch STREAMSIX website on the external browser.
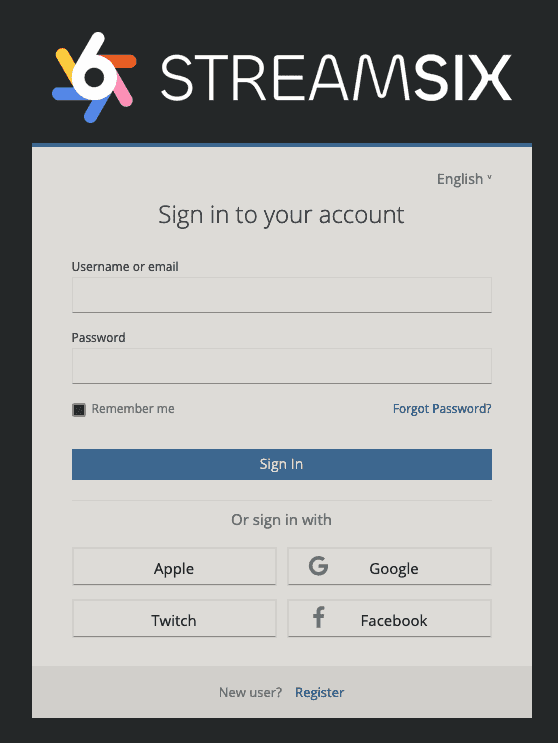
From the website, user has various options to login. Either by using their STREAMSIX username and password or through a 3rd party OIDC.
For platforms that support deeplinking, the login process can be redirected from the browser back to the game by using the AuthController.LoginExternalWebpageWithDeepLinkCallback()
.
Login through STREAMSIX by username and password
Call AuthController.LoginWithEmailAsync
to login directly in the game. This is not recommended as this feature may be removed in the future as it gives non affiliate games a way of password phishing the user-base.
Login to 3rd party OIDC provider
Direct login in the browser
An optional Provider
enum value may be provided to either of the aforementioned login methods AuthController.LoginExternalWebpage()
or AuthController.LoginExternalWebpageWithDeepLinkCallback()
to explicitly jump to logging in to STREAMSIX with the provided OIDC provider. For example, AuthController.LoginExternalWebpage(Provider.Twitch)
will automatically redirect to twitch and redirect back to keycloak once logged in (and authorized if this is the first time logging in to STREAMSIX with this account). If the Twitch account doesn't have a linked STREAMSIX account an account will be created for them and they will be logged in.
For apple login on iOS devices to conform to Apple's guidlines, it should be done natively. For details, see the example apple login sample in the unity package.
Note: This is the prefered way to login with an external OIDC provider
Linking accounts
To link an already logged in STREAMSIX account to an OIDC, AuthController.LinkIdpExternalWebpage(<oidc-provider>)
or AuthController.LinkIdpExternalWebpageWithDeepLinkCallback(<provider>)
can be used where the OIDC provider is one of the Provider
enum values. Once the linking has been completed, the tokens will be refreshed and the OIDC provider id an username will be present.
Example:
AuthController.LinkIdpExternalWebpage(Provider.Twitch)
Twitch specific permissions
A game may need specific permissions from an OIDC provider for gameplay (for example, to access Twitch chat data). TwitchController
is provided for this use. Once user is logged in, the function returns the access/refresh tokens from Twitch. Whether deep link is setup or not, this function always return the tokens from Twitch unless it ran into an error. The game should store this info locally if it needs to access Twitch API later. The SDK does not store this info.
twitchData = await TwitchController.LoginToTwitch(License, ClientID);
Setup Deep Link
Add AuthDeepLink
monobehaviour to your scene. Set Application Scheme
to a name unique to your game.
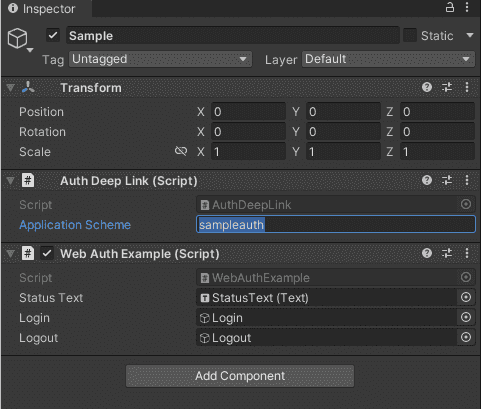
When AuthController.InitAsync()
is called, it will search for this monobehaviour. Be sure that it is present in the scene at that time.
For android platform, follow Unity documentation to create a file at Assets/Plugins/Android/AndroidManifest.xml https://docs.unity3d.com/Manual/deep-linking-android.html
There are two deep link that login use:
<data android:scheme="unitydl" android:host="login" /><data android:scheme="unitydl" android:host="logout" />
Add both of these to the xml file.
Replace unitydl
in the xml with your application scheme defined in AuthDeepLink MonoBehaviour above.